Order parameter (GL)
The order parameter specifies how to
arrange the nodes.
Example of specifying node placement
iterations and allowed time (GL algorithm)
To specify the ordering option for the
nodes:
In CSS
Add to the GraphLayout section:
nodeComparator : "DESCENDING_HEIGHT";
In Java™
Use the method:
void setNodeComparator(Comparator comparator)
The order parameter specifies how to
arrange the nodes.
The valid values for
comparator
are:
AUTOMATIC_ORDERING
The algorithm is free to choose the order in such a way that it tries to reduce the total area occupied by the layout.NO_ORDERING
No ordering is performed.DESCENDING_HEIGHT
The nodes are ordered in the descending order of their height.ASCENDING_HEIGHT
The nodes are ordered in the ascending order of their height.DESCENDING_WIDTH
The nodes are ordered in the descending order of their width.ASCENDING_WIDTH
The nodes are ordered in the ascending order of their width.DESCENDING_AREA
The nodes are ordered in the descending order of their area.ASCENDING_AREA
The nodes are ordered in the ascending order of their area.
- Any other implementation of the
java.util.Comparator
interface.The nodes are ordered according to this custom comparator.
The default is
AUTOMATIC_ORDERING
.
In incremental mode (see setIncrementalModesetIncrementalMode) and with fixed nodes
(see setFixedsetFixed), the order of the nodes is not
completely preserved.
If the layout mode is TILE_TO_GRID_FIXED_WIDTHTILE_TO_GRID_FIXED_WIDTH or TILE_TO_GRID_FIXED_HEIGHTTILE_TO_GRID_FIXED_HEIGHT, the order
options are applied only for nodes whose size (including
margins) is smaller than the grid cell size (see setHorizontalGridOffsetsetHorizontalGridOffset and setVerticalGridOffsetsetVerticalGridOffset).
Layout modes (GL)
The Grid Layout algorithm has four layout
modes.
Example of selecting a layout mode (GL
algorithm)
To select a layout mode:
In CSS
Add to the
GraphLayout
section:
layoutMode : "TILE_TO_GRID_FIXED_HEIGHT";
In Java
Use the method:
void setLayoutMode(int mode);
The valid values for
mode
are:
IlvGridLayout.TILE_TO_GRID_FIXED_WIDTH
(the default).The nodes are placed in the cells of a grid (matrix) that has a fixed maximum number of columns. This number is equal to the width of the layout region parameter divided by the horizontal grid offset.IlvGridLayout.TILE_TO_GRID_FIXED_HEIGHT
The nodes are placed in the cells of a grid (matrix) that has a fixed maximum number of rows. This number is equal to the height of the layout region parameter divided by the vertical grid offset.IlvGridLayout.TILE_TO_ROWS
The nodes are placed in rows. The maximum width of the rows is equal to the width of the layout region parameter. The height of the row is the maximum height of the nodes contained in the row (plus margins).IlvGridLayout.TILE_TO_COLUMNS
The nodes are placed in columns. The maximum height of the columns is equal to the height of the layout region parameter. The width of the column is the maximum width of the nodes contained in the column (plus margins).
Alignment parameters (GL)
Global alignment parameters
The alignment options control how a node
is placed over its grid cell or over its row or column
(depending on the layout mode). The alignment can be set
globally, in which case all nodes are aligned in the same way,
or locally on each node, with the result that different
alignments occur in the same drawing.
Example of setting global alignment
(GL algorithm)
To set the global alignment:
In CSS
Add to the
GraphLayout
section:
globalHorizontalAlignment : "LEFT"; globalVerticalAlignment : "TOP";
In Java
Use the following methods:
void setGlobalHorizontalAlignment(int alignment);
void setGlobalVerticalAlignment(int alignment);
The valid values for the alignment
parameter are:
IlvGridLayout.CENTER
(the default)The node is horizontally and vertically centered over its grid cell or row or column.IlvGridLayout.TOP
The node is vertically aligned on the top of its cell or row. Not used if the layout mode isTILE_TO_COLUMNS
.IlvGridLayout.BOTTOM
The node is vertically aligned on the bottom of its grid cell or row. Not used if the layout mode isTILE_TO_COLUMNS
.IlvGridLayout.LEFT
The node is horizontally aligned on the left of its grid cell or column. Not used if the layout mode isTILE_TO_ROWS
.IlvGridLayout.RIGHT
The node is horizontally aligned on the right of its grid cell or column. Not used if the layout mode isTILE_TO_ROWS
.IlvGridLayout.MIXED
Each node can have a different alignment. The alignment of each individual node can be set with the result that different alignments can occur in the same graph.
Alignment of individual nodes
All nodes have the same alignment unless the global alignment
is set to
IlvGridLayout.MIXED
. Only when the global alignment is set to mixed can each
node have an individual alignment style.
Example of setting alignment of
individual nodes (GL algorithm)
To set and retrieve the alignment of
an individual node:
In CSS
Write a rule that selects the node,
for instance:
GraphLayout { globalVerticalAlignment : "MIXED"; } #node1{ VerticalAlignment : "BOTTOM"; }
In Java
Use the following methods:
void setHorizontalAlignment(Object node, int alignment);
void setVerticalAlignment(Object node, int alignment);
int getHorizontalAlignment(Object node);
int getVerticalAlignment(Object node);
The valid values for the alignment
parameter are:
IlvGridLayout.CENTER
(the default)IlvGridLayout.TOP
IlvGridLayout.BOTTOM
IlvGridLayout.LEFT
IlvGridLayout.RIGHT
Maximum number of nodes per row or column (GL)
By default, in
IlvGridLayout.TILE_TO_ROWS
or
IlvGridLayout.TILE_TO_COLUMNS
mode, the layout places as many nodes on each row or column as
possible given the size of the nodes and the dimensional
parameters (layout region and margins). If needed, the layout
can additionally respect a specified maximum number of nodes
per row or column.
Example of specifying the maximum number
of nodes per row or column (GL algorithm)
To set the maximum number of nodes per
row or column:
In CSS
Add to the
GraphLayout
section:
maxNumberOfNodesPerRowOrColumn : "8";
In Java
Use the method:
void setMaxNumberOfNodesPerRowOrColumn(int nNodes);
The default value is
Integer.MAX_VALUE
, that is, the number of nodes placed in each row or column is
bounded only by the size of the nodes and the dimensional
parameters. The specified value must be at least
1
. The parameter has no effect if the layout mode is
IlvGridLayout.TILE_TO_GRID_FIXED_WIDTH
or
IlvGridLayout.TILE_TO_GRID_FIXED_HEIGHT
.
Incremental mode (GL)
The Grid Layout algorithm normally places
all the nodes from scratch. If the graph incrementally changes
because you add, remove, or resize nodes, the subsequent layout
can differ considerably from the previous layout. To avoid this
effect and to help the user to retain a mental map of the graph,
the algorithm has an incremental mode. In incremental mode, the
layout tries to place the nodes at the same location or in the
same order as in the previous layout whenever it is possible.
Example of enabling the incremental mode
(GL algorithm)
To enable the incremental mode:
In CSS
Add to the
GraphLayout
section:
incrementalMode : "true";
Note
To preserve stability, the incremental
mode can keep some regions free. Therefore, the total area of
the layout can be larger than in nonincremental mode, and, in
general, the layout cannot look as nice as in nonincremental
mode.
Dimensional parameters (GL)
Dimensional parameters for the grid mode of
the Grid Layout algorithm and Dimensional parameters for the
row-and-column mode of the Grid Layout algorithm illustrate the
dimensional parameters used in the Grid Layout algorithm. These
parameters are explained in the sections that follow.
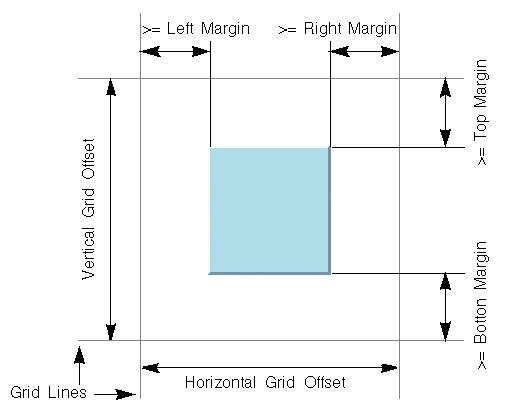
Dimensional parameters for the grid
mode of the Grid Layout algorithm

Dimensional parameters for the
row-and-column mode of the Grid Layout algorithm
Grid offset (GL)
The grid offset parameters control the spacing between grid
lines. It is taken into account only by the grid mode (layout
modes
TILE_TO_GRID_FIXED_WIDTH
and
TILE_TO_GRID_FIXED_HEIGHT
).
Example of setting grid offset (GL
algorithm)
To set the horizontal and vertical grid
offset:
In CSS
Add to the
GraphLayout
section:
horizontalGridOffset : "50.0";
verticalGridOffset : "70.0";
In Java
Use the methods:
void setHorizontalGridOffset(float offset);
void setVerticalGridOffset(float offset);
The grid offset is the critical parameter
for the grid mode. If the grid offset is larger than the size of
the nodes (plus margins), an empty space is left around the node.
If the grid offset is smaller than the size of the nodes (plus
margins), the node must be placed on more than one grid cell. The
best choice for the grid offsets depends on the application. It
can be computed according to either the maximum size of the nodes
(plus margins) or the medium size, and so on. If all the nodes
have a similar size, the choice is straightforward.
Margins (GL)
The margins control the space around each
node that the layout algorithm keeps empty.
Example of specifying margins (GL
algorithm)
To set the margins:
In CSS
Add to the
GraphLayout
section:
topMargin : "6.0"; bottomMargin : "6.0"; leftMargin : "4.0"; rightMargin : "4.0";
In Java
Use the methods:
void setTopMargin(float margin);
void setBottomMargin(float margin);
void setLeftMargin(float margin);
void setRightMargin(float margin);
The meaning of the margin parameters is
not the same for the grid modes as for the row/column modes:
- In grid modes, they represent the minimum distance between the node border and the grid line (see Dimensional parameters for the grid mode of the Grid Layout algorithm.)
- In row/column modes, they are used to control the vertical distance between the rows or the horizontal distance between the columns and the horizontal or vertical minimum distance between the nodes in the same row or column (see Dimensional parameters for the row-and-column mode of the Grid Layout algorithm).
The default value for all the margin parameters is
5
.